Trade Execution
On our platform, we offer users the ability to execute trades through two main order types: Limit Orders and Market Orders. Each order type serves a specific purpose and has advantages and disadvantages for traders.
Limit order strategy
A Limit Order is an order to buy or sell an asset at the best market price. A Limit Order allows traders to set a specific price at which they are willing to buy or sell an asset. When the market price reaches the specified limit price, the Limit Order is executed, and the trade is executed. Limit Orders provide greater control over trading activities and allow traders to obtain better prices by buying low or selling high.
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.cyan.test.krayondigital.com/main/api/v1/trades',
headers: {
accept: 'application/json',
'content-type': 'application/json',
'Bearer': 'API-TOKEN'
},
data: {
"ticker": "ETH-USDC",
"side": "BUY",
"delivered_amount": 100,
"strategy": "limit",
"wallet": "{{ID_WALLET_ID}}"
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.cyan.test.krayondigital.com/main/api/v1/trades"
payload = {
"ticker": "ETH-USDC",
"side": "BUY",
"delivered_amount": 100,
"strategy": "limit",
"wallet": "{{ID_WALLET_ID}}"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
"Bearer": "API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
Market order strategy
A Market Order is an order to buy or sell an asset at the current market price. When a Market Order is placed, the transaction is executed immediately. Market Orders are suitable for traders prioritizing speed and efficiency over accurate pricing. However, those orders do not always provide the best price, especially in volatile markets where prices fluctuate rapidly.
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.cyan.test.krayondigital.com/main/api/v1/trades',
headers: {
accept: 'application/json',
'content-type': 'application/json',
'Bearer': 'API-TOKEN'
},
data: {
"ticker": "ETH-USDC",
"side": "BUY",
"delivered_amount": 100,
"strategy": "market",
"wallet": "{{ID_WALLET_ID}}"
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.cyan.test.krayondigital.com/main/api/v1/trades"
payload = {
"ticker": "ETH-USDC",
"side": "BUY",
"delivered_amount": 100,
"strategy": "market",
"wallet": "{{ID_WALLET_ID}}"
}
headers = {
"accept": "application/json",
"content-type": "application/json"
"Bearer": "API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
Privacy order strategy - New
This strategy allows users to send ERC20/ETH tokens from one address to another without connecting the two addresses through on-chain transactions. This provides an additional privacy and security for users who prioritize anonymity.
To issue a Privacy order, users simply select the type of trade when sending tokens from our platform. The platform will then execute the trade on their behalf, ensuring a seamless and secure transaction.
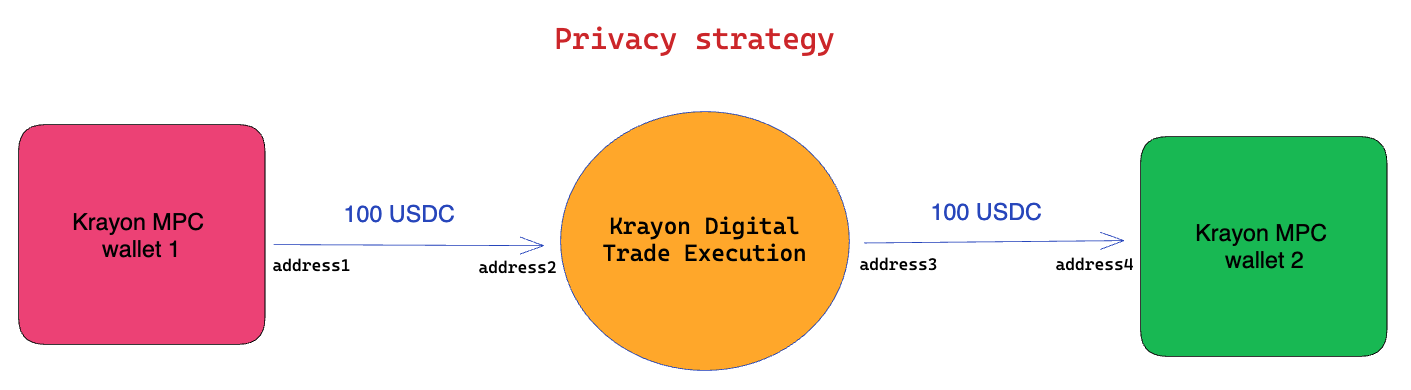
Privacy Strategy
Flow:
- The user opens two MPC wallets in the Krayon Digital platform.
- The user calls the Trade API to send supported trading ETH/ERC20 tokens supported-coins .
- ETH/ERC20 tokens are moved from MPC wallet 1(address 1) to Krayon
Trade Execution address (address 2). Then the ETH/ERC20 automatically moves from
Krayon (address 3) to the MPC wallet 2 (address 4).
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.cyan.test.krayondigital.com/main/api/v1/trades',
headers: {
accept: 'application/json',
'content-type': 'application/json',
'Bearer': 'API-TOKEN'
},
data: {
"delivered_amount": 10,
"deliver_currency": "ETH",
"strategy": "BLENDER",
"wallet": "{{SRC_WALLET_ID}}", # id(not address) of MPC wallet 1
"destination_wallet": "{{DST_WALLET_ID}}" # id(not address) of MPC wallet 2
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.cyan.test.krayondigital.com/main/api/v1/trades"
payload = {
"delivered_amount": 10,
"deliver_currency": "ETH",
"strategy": "BLENDER",
"wallet": "{{SRC_WALLET_ID}}", # id(not address) of MPC wallet 1
"destination_wallet": "{{DST_WALLET_ID}}" # id(not address) of MPC wallet 2
}
headers = {
"accept": "application/json",
"content-type": "application/json"
"Bearer": "API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
Updated 5 months ago