Crypto Deposits
Learn how to generate crypto deposits
Introduction
This guide provides a comprehensive overview of how to integrate the new "Crypto Deposits" feature into your platform. This feature allows clients to create derivative wallets from a master wallet, generate crypto deposits, and automate the sweeping of wallets based on specific criteria.
Prerequisites
As described in the Deposits API and API Endpoints documentation, you need to know Krayon APIs and understand wallet operations and cryptocurrency transactions.
To use the Crypto Deposit feature, you must create a master wallet from which all derivative wallets can be created.
Process Flow
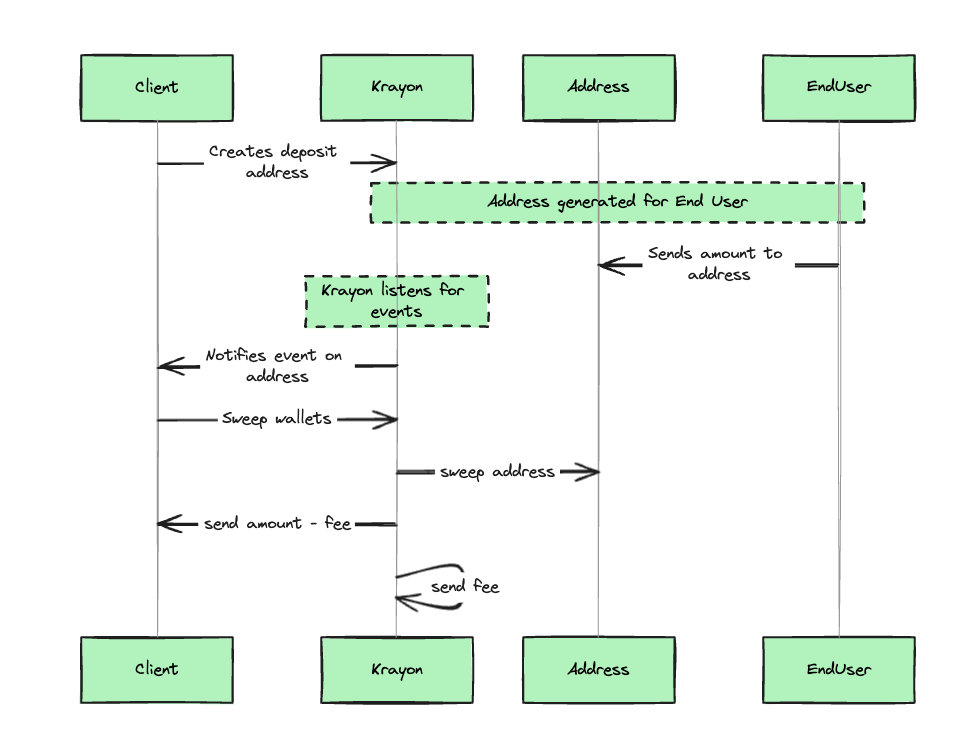
Step 1: Creating a Derivative Wallet From a Master Wallet
Purpose: Generate a unique wallet address for the end user
Process: Use the Krayon Digital API to create a derivative wallet from your master wallet. Create derived wallet.
See the "Generate Derived Wallet" section on this page.
Step 2: Generating a Crypto Deposit
Purpose: Create a deposit transaction for cryptocurrencies like USDT or USDC.
Process: Initiate a crypto deposit using the Krayon Digital API. Create deposit.
See the "Creating a Deposit" section on this page.
Step 3: Notifying the Client
Purpose: Inform the client when a transaction is detected in the derived wallet.
Process: Set webhook notifications to notify the client when a transaction is confirmed. Create subscription.
See details on setting up webhooks here.
Step 4: Sweeping the Wallets
Purpose: Consolidate funds from derivative wallets back into the master wallet.
Triggers: Set triggers based on cryptocurrency symbol, blockchain network, minimum amount, and a predefined schedule. You can initiate a sweeping process using the Krayon Digital API. Create settlement..
See the "Sweeping the wallets" section for implementation instructions.
Generate Crypto Deposit - Detailed
When generating a deposit request, the system initiates the deposit creation and redirects the user to the provided crypto payment link.
You must provide all the necessary information to facilitate the completion of the deposit request. Upon receipt of this information, our system will respond with payment metadata, which includes an external link for the user to access and view their payment details.
Note: Failure to provide all required fields in your request will result in an error response from the Krayon API endpoint.
Create A master Wallet
Request:
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.aqua.test.krayondigital.com/main/api/v1/wallets',
headers: {
'accept': 'application/json',
'content-type': 'application/json',
'Authorization': "Bearer YOUR-API-TOKEN"
},
data: {
"name": "Master Wallet",
"blockchain": "tron" //ethereum, bsc, etc.
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.aqua.test.krayondigital.com/main/api/v1/wallets"
payload = {
"name": "Master Wallet",
"blockchain": "tron" # ethereum, bsc, etc.
}
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "Bearer YOUR-API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
Response:
{
"data": {
"id": "224d57de3c9842e5af2e89bd36e6bdc9",
"total_usd_balance": 0,
"pending_usd_balance": 0,
"address": "TAH8FwdSKLkKfKqR3ZLwNhcrUEHKmc7zXK",
"organization": "org_aAgoWL5DjwSvQSf0",
"blockchain": "tron",
"num_quorum": 1,
"gas_station_status": 0,
"name": "Master Wallet",
"group": null,
"type": "NATIVE",
"description": "",
"image": "",
"parent": null,
"available_usd_balance": 0
}
}
Create cluster wallets
You need to create different cluster wallets from the Master Wallet, allowing you to sweep deposits on networks such as Tron, Binance Smart Chain, and Ethereum.
If the first master wallet was created on the Tron blockchain, you cannot create another cluster wallet on that blockchain; you need to add the Binance Smart Chain and Ethereum blockchains manually.
This can be done in two ways:
Dashboard
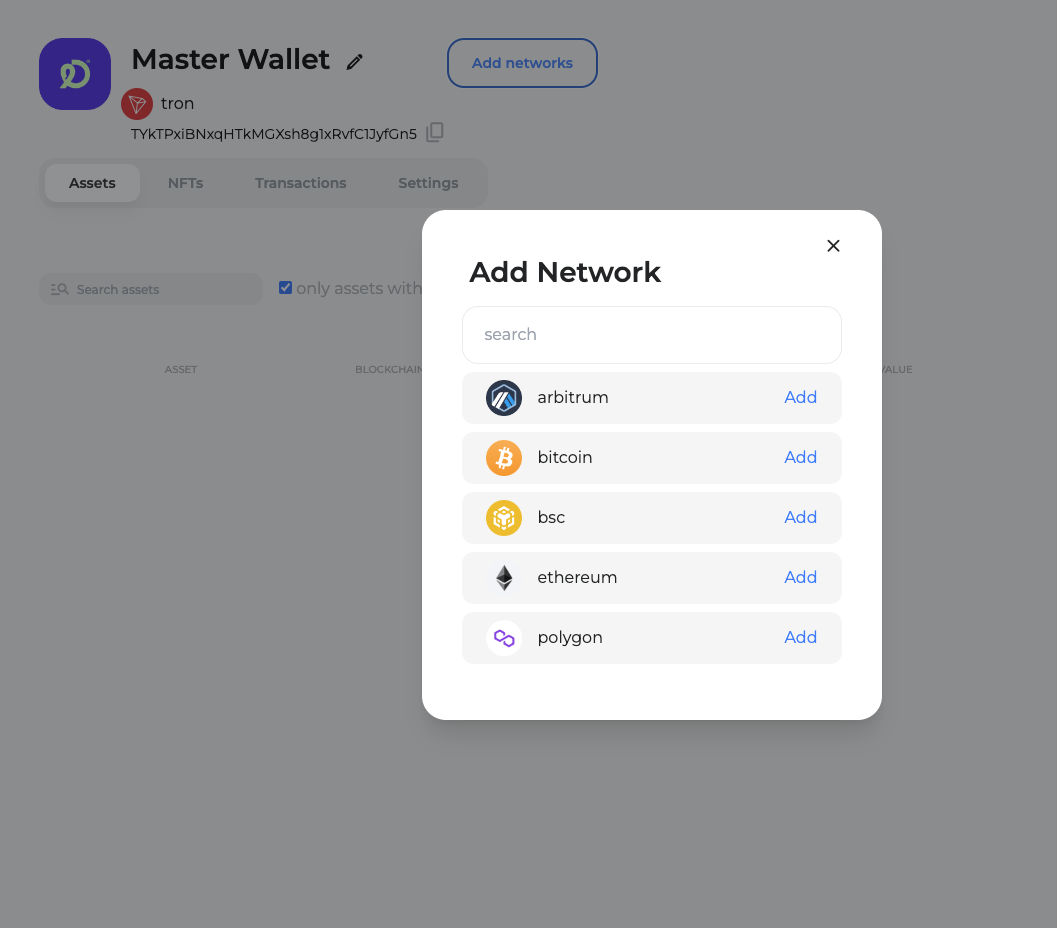
Add Cluster Wallet
API Request:
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.aqua.test.krayondigital.com/main/api/v1/wallets',
headers: {
'accept': 'application/json',
'content-type': 'application/json',
'Authorization': "Bearer YOUR-API-TOKEN"
},
data: {
"blockchain": "bsc",
"group": null,
"parent": "224d57de3c9842e5af2e89bd36e6bdc9"
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.aqua.test.krayondigital.com/main/api/v1/wallets"
payload = {
"blockchain": "bsc",
"group": null,
"parent": "224d57de3c9842e5af2e89bd36e6bdc9"
}
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "Bearer YOUR-API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
- You need to use the master wallet id from last call to create the cluster wallet on BSC blockchain.
Generate Derived Wallet
You need to take the ID of the master wallet from the example above, 224d57de3c9842e5af2e89bd36e6bdc9, and use it in the next API call. Even though the master wallet is on the Tron blockchain, you can create any derivative wallet on other blockchains than the master wallet. Currently, we only support Ethereum, Tron, and BSC. It is recommended to use different master wallets for other blockchains, to make a simple separation between the networks.
Request:
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.aqua.test.krayondigital.com/main/api/v1/derived-wallets',
headers: {
'accept': 'application/json',
'content-type': 'application/json',
'Authorization': "Bearer YOUR-API-TOKEN"
},
data: {
"parent": "224d57de3c9842e5af2e89bd36e6bdc9",
"blockchain": "tron" //ethereum, bsc, etc.
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import axios from 'axios';
const options = {derived-wallets
method: 'POST',
url: 'https:/api.aqua.test.krayondigital.com/main/api/v1/derived-wallets',
headers: {
'accept': 'application/json',
'content-type': 'application/json',
'Authorization': "Bearer YOUR-API-TOKEN"
},
data: {
"parent": "224d57de3c9842e5af2e89bd36e6bdc9",
"blockchain": "tron" //ethereum, bsc, etc.
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
Response (tron):
{
"data": {
"id": "272e30b7e9b24c48b0b2664e4c3d4803",
"total_usd_balance": 0,
"pending_usd_balance": 0,
"address": "TV79Zd1wPq2zssHm8SJAiXYGy5abjT4STy",
"organization": "org_aAgoWL5DjwSvQSf0",
"blockchain": "tron",
"num_quorum": 1,
"gas_station_status": 0,
"name": "Master Wallet - tron - m/0/0/1",
"group": null,
"type": "NATIVE",
"description": "",
"image": "",
"parent": "224d57de3c9842e5af2e89bd36e6bdc9",
"available_usd_balance": 0
}
}
Resposne(ethereum, 2nd derived wallet)
{
"data": {
"id": "3f7c85d461294a328c3a52bb1af64316",
"total_usd_balance": 0,
"pending_usd_balance": 0,
"address": "0x96d4f00dff1e1d657e9e27a5e37e75e8c783bbd2",
"organization": "org_aAgoWL5DjwSvQSf0",
"blockchain": "ethereum",
"num_quorum": 1,
"gas_station_status": 0,
"name": "Master Wallet - ethereum - m/0/0/2",
"group": null,
"type": "NATIVE",
"description": "",
"image": "",
"parent": "224d57de3c9842e5af2e89bd36e6bdc9",
"available_usd_balance": 0
}
}
- m/0/0/2 is the path of the derived wallet. This is a running counter of derivative wallet numbers. The master wallet path is m/0/0/0.
Create a Crypto Deposit
You need to use the wallet ID you have previously created. The crypto deposits blockchain is derived from the Derived Wallet blockchain.
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.aqua.test.krayondigital.com/main/api/v1/deposits',
headers: {
'accept': 'application/json',
'content-type': 'application/json',
'Authorization': "Bearer YOUR-API-TOKEN"
},
data: {
"amount": 100,
"reference_id": "crypto_payment_1",
"description": "this is the crypto payment description",
"customer": {
"country_code": "US",
"email": "[email protected]"
},
"payment_method": "CRYPTO",
"symbol": "USDC",
"type":"DEPOSIT",
"wallet": "272e30b7e9b24c48b0b2664e4c3d4803",
"webhook_url":"https://eo3y4clrcxypoxe.m.pipedream.net"
}
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.aqua.test.krayondigital.com/main/api/v1/deposits"
payload = {
"amount": 100,
"reference_id": "crypto_payment_1",
"description": "this is the crypto payment description",
"customer": {
"country_code": "US",
"email": "[email protected]"
},
"payment_method": "CRYPTO",
"symbol": "USDC",
"type":"DEPOSIT",
"wallet": "272e30b7e9b24c48b0b2664e4c3d4803",
"webhook_url":"https://eo3y4clrcxypoxe.m.pipedream.net"
}
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "Bearer YOUR-API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
Sweeping the Wallets
You need to use the Master Wallet ID you previously created. Then issue a settlement request with the symbol to sweep across all blockchains. After creating the request, the sweep process will run in the background.
import axios from 'axios';
const options = {
method: 'POST',
url: 'https:/api.aqua.test.krayondigital.com/main/api/v1/settlements',
headers: {
'accept': 'application/json',
'content-type': 'application/json',
'Authorization': "Bearer YOUR-API-TOKEN"
},
data: {
"amount": 10,// Optional
"symbol": "USDC",
"payment_method": "CRYPTO",
"wallet": "dff2717a2430427da6d00ebde5042a06", //this is the master wallet's id
"description": "A Settlement Description"
};
axios
.request(options)
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.error(error);
});
import requests
url = "https:/api.aqua.test.krayondigital.com/main/api/v1/settlements"
payload = {
"amount": 10, # Optional
"symbol": "USDC",
"payment_method": "CRYPTO",
"wallet": "dff2717a2430427da6d00ebde5042a06", # this is the master wallet's id
"description": "A Settlement Description"
};
headers = {
"accept": "application/json",
"content-type": "application/json",
"Authorization": "Bearer YOUR-API-TOKEN"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
You can always check the current status of the settlement here or in the dashboard as well.
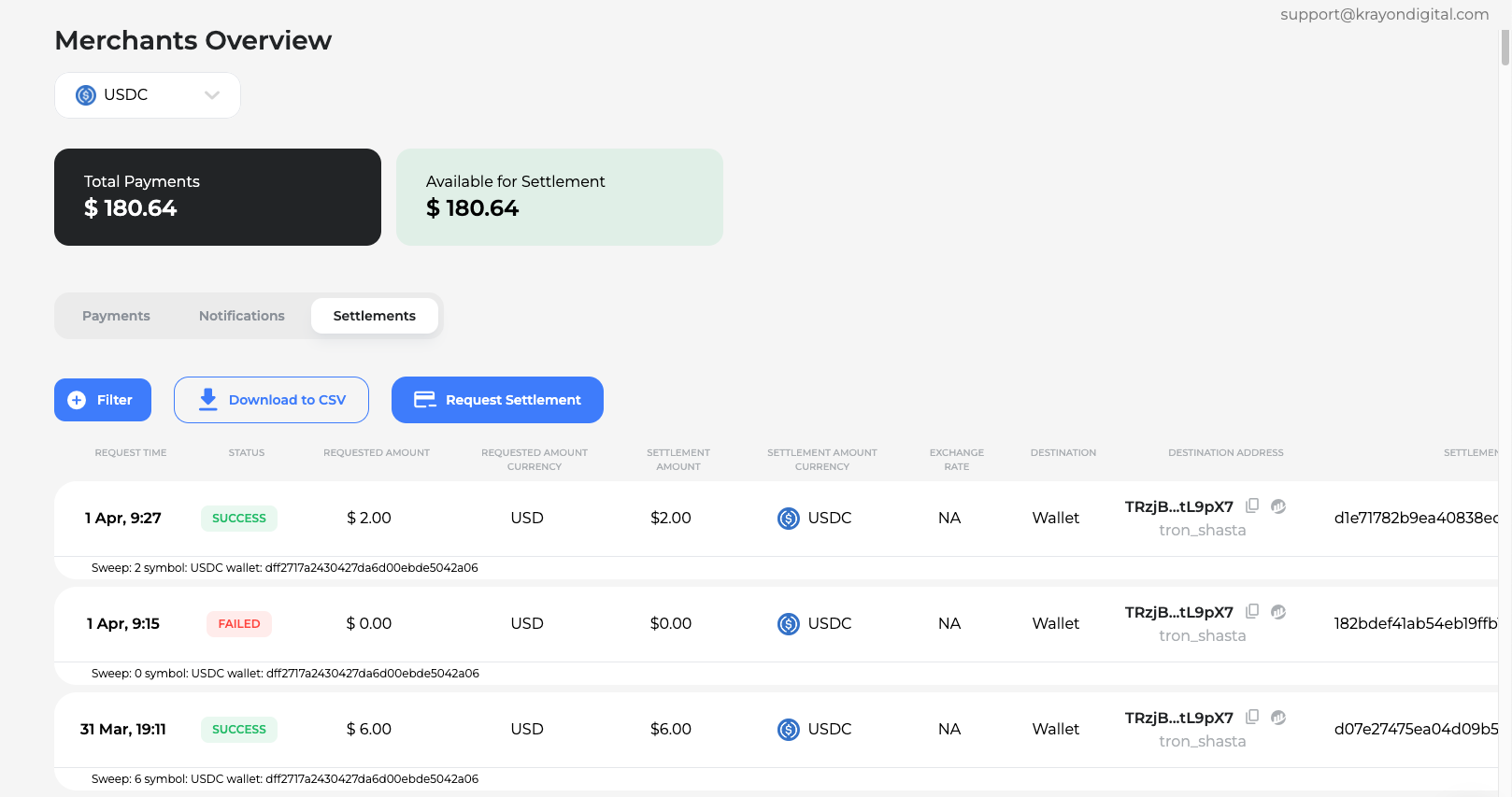
Merchants Settlements View
Updated 9 days ago